UI Customization
The UICustomization class exposes functionality to customize the 3DS SDK UI elements. This allows the 3DS Requestor application to have its own look-and-feel in the native challenge pages. The customization of Buttons, Labels, TextBoxes, and Toolbars is enabled via the various classes and subclasses outlined below.
UICustomization
Main class for configuring the customization of UI elements.
public class UiCustomization {
enum ButtonType (VERIFY, CONTINUE, NEXT, CANCEL, RESEND)
enum LabelType (INFO_HEADER, INFO_TEXT, INFO_LABEL, WHITELIST, WHY_INFO, WHY_INFO_TEXT, EXPANDABLE_INFO, EXPANDABLE_INFO_TEXT, SELECTION_LIST)
void setButtonCustomization(ButtonCustomization buttonCustomization, ButtonType buttonType)
void setToolbarCustomization(ToolbarCustomization toolbarCustomization)
void setLabelCustomization(LabelCustomization labelCustomization)
void setTextBoxCustomization(TextBoxCustomization textboxCustomization)
void setBackground(UIColor color)
void setInformationZoneIconPosition(int position) // 0: right, 1: left, 2: hide
void setBrandingZoneLogoGap(CGFloat value)
ButtonCustomization getButtonCustomization(ButtonType buttonType)
ToolbarCustomization getToolbarCustomization()
LabelCustomization getLabelCustomization()
TextBoxCustomization getTextBoxCustomization()
}
ButtonCustomization
The ButtonCustomization class enables the customization of various buttons presented to the cardholder during the challenge process.
public class ButtonCustomization {
void setBackgroundColor(UIColor color)
void setCornerRadius(int cornerRadius)
void setTextFontName(String fontName)
void setTextFontSize(int fontSize)
void setTextColor(String hexColorCode)
void setTextColor(UIColor color)
void setHeight(CGFloat height)
void setPadding(UIEdgeInsets edge) // applicable only for ButtonType of CANCEL
UIColor getBackgroundColor()
int getCornerRadius()
String getTextFontName()
int getTextFontSize()
UIColor getTextColor()
String getTextColorHex()
CGFloat getHeight()
}
For example, the following code will configure buttons with red backgrounds and white font:
let uiCustom = UiCustomization()
let customButton = ButtonCustomization()
customButton.setTextColor(color: UIColor.white)
customButton.setBackgroundColor(color:UIColor.red)
try uiCustom.setButtonCustomization(buttonCustomization: customButton, buttonType: .CANCEL)
try uiCustom.setButtonCustomization(buttonCustomization: customButton, buttonType: .CONTINUE)
try uiCustom.setButtonCustomization(buttonCustomization: customButton, buttonType: .NEXT)
try uiCustom.setButtonCustomization(buttonCustomization: customButton, buttonType: .RESEND)
try uiCustom.setButtonCustomization(buttonCustomization: customButton, buttonType: .SUBMIT)
This results in the following Submit button for a Single-Select challenge:
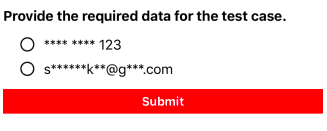
LabelCustomization
The LabelCustomization class enables the customization of various labels displayed throughout the challenge process.
public class LabelCustomization {
void setHeadingTextAlignment(NSTextAlignment textAlignment)
void setHeadingTextColor(UIColor color)
void setHeadingTextFontName(String fontName)
void setHeadingTextFontSize(int fontSize)
void setTextColor(String hexColorCode)
void setTextColor(UIColor color)
void setTextColor(LabelType labelType, UIColor color)
void setTextFontName(String fontName)
void setTextFontName(LabelType labelType, String fontName)
void setTextFontSize(int fontSize)
void setTextFontSize(LabelType, labelType, int fontSize)
void setBackgroundColor(LabelType labelType, UIColor color)
void setPadding(LabelType LabelType, UIEdgeInsets edge)
NSTextAlignment getHeadingTextAlignment()
UIColor getHeadingTextColor()
String getHeadingTextFontName()
int getHeadingTextFontSize()
UIColor getTextColor()
String getTextColorHex()
String getTextFontName()
int getTextFontSize()
}
TextBoxCustomization
The TextBoxCustomization class enables the customization of textboxes displayed throughout the challenge process.
public class TextBoxCustomization {
void setBorderColor(UIColor color)
void setBorderWidth(int borderWidth)
void setCornerRadius(int cornerRadius)
void setTextColor(String hexColorCode)
void setTextColor(UIColor color)
void setTextFontName(String fontName)
void setTextFontSize(int fontSize)
UIColor getBorderColor()
int getBorderWidth()
int getCornerRadius()
UIColor getTextColor()
String getTextColorHex()
String getTextFontName()
int getTextFontSize()
}
ToolbarCustomization
The ToolbarCustomization class enables the customization of the toolbar displayed throughout the challenge process.
public class ToolbarCustomization {
void setBackgroundColor(UIColor color)
void setButtonText(String buttonText)
void setHeaderText(String headerText)
void setTextColor(String hexColorCode)
void setTextColor(UIColor color)
void setTextFontName(String fontName)
void setTextFontSize(int fontSize)
UIColor getBackgroundColor()
String getButtonText()
String getHeaderText()
UIColor getTextColor()
String getTextColorHex()
String getTextFontName()
int getTextFontSize()
}